Unity Collision Detection in 25 Seconds
Article Purpose
The purpose of this article is to showcase a simple approach for doing collision detection in Unity without the GameObject
collisions impacting each other with physics.
There are numerous ways to do collision detection in general, but this is the simplest way in Unity using Unity's built-in components.
The Setup
We are going to keep this example extremely small to highlight just the essential elements. For this approach to work, you need to use three components. The first two are Unity specific and the third is a custom component:
RigidBody
component- Collider component (
BoxCollider
,SphereCollider
,CapsuleCollider
, orMeshCollider
) - Custom Script component implementing any combination of the
OnTriggerEnter()
,OnTriggerStay()
, orOnTriggerExit()
methods
Take note that there are 2D equivalents to all the aforementioned Unity specific components and OnTrigger()
methods, you simply append 2D to their name.
Game Time
Now that we know the components we’re going to be working with, let’s set up a simple example. This step-by-step breakdown assumes you have opened a new scene that contains only the Main Camera that Unity automatically sets up. In addition you’ll want to have added this CollisionScript
component to your Project:
using UnityEngine;
using System.Collections;
public class CollisionScript : MonoBehaviour {
private void OnTriggerEnter(Collider that) {
Debug.Log(this + " entered " + that);
}
}
Now simply follow along to these steps and you’ll be up and running.
- Add a Cube and a Sphere to the scene (using Unity’s default 3D objects)
- By default, they will have a
BoxCollider
andSphereCollider
component respectively, check the IsTrigger checkbox of each - Multi-select the Cube and the Sphere and add a
RigidBody
component to both at the same time - With both still selected, uncheck Use Gravity and check Is Kinematic
- With both still selected, drag and drop the
CollisionScript
into the Inspector - Press Play
Here's a GIF showing the above steps in action:
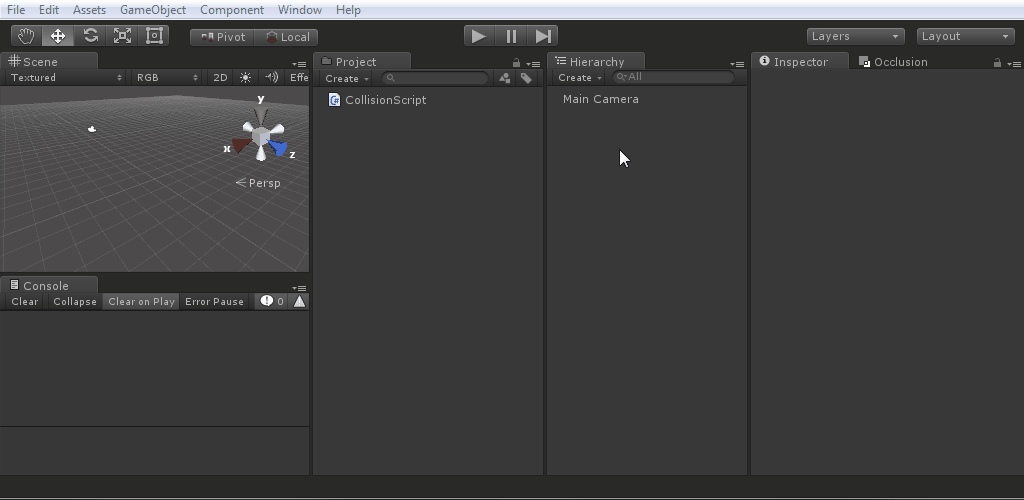
You’ll notice that the Console logs two messages, one for the Cube and one for the Sphere. You can select just one of the objects now and use the Transform Gizmo to simulate additional collisions.
Conclusion
This is a basic example, but it illustrates the simple relationship between a RigidBody
that doesn’t use gravity and a Collider that uses triggers. The custom CollisionScript
is something you’ll want to expand on. Most likely you’ll want the OnTrigger()
methods to dispatch a custom event that holds references to both objects involved in the collision. Then you could have an event listener analyze the objects to determine what to do next. Happy colliding.